Overview
Integrating with the Extend SDK will allow you to display offers and sell extended warranty contracts in your online store. This guide will walk you through how to do the following:
- Set up your store with Extend
- Add the Extend SDK scripts
- Render Extend offer buttons on your product display page
- Add the Extend offer modal and add warranties to the cart
- Extend Shipping Insurance Cart Integration
- Handle multiple product variants
- Add extended warranties to your cart
- Cart normalization and quantity matching
- Support post-purchase warranty functionality
- ExtendShopify API reference
Before you start: Make sure you have installed the Shopify Extend App. Visit merchants.extend.com and login with your Shopify account.
Set up your store with Extend
Before you start integrating with the Extend SDK you’ll need to do the following:
Create a Copy of Your Current Theme
From the Shopify admin, go to Online Store → Themes → Actions → Duplicate
Get your store ID
In order to configure the SDK to your store, you will need your Store ID.
Go to merchants.extend.com→ Settings → Production/Sandbox credentials
Add the Extend SDK scripts
Add the following scripts into your theme.liquid file right before the closing </head>
tag and press save.
<script src="https://sdk.helloextend.com/extend-sdk-client/v1/extend-sdk-client.min.js"></script>
<script src="https://sdk.helloextend.com/extend-sdk-client-shopify-addon/v1/extend-sdk-client-shopify-addon.min.js"></script>
<script>
Extend.config({
storeId: '<YOUR_EXTEND_STORE_ID>',
environment: 'production',
})
</script>
<script>
Extend.config({ storeId: '<YOUR_EXTEND_STORE_ID>', environment: 'demo' })
</script>
To verify the scripts are running correctly, preview your theme copy, open your browser’s console, and type ‘Extend’ or ‘ExtendShopify’ and hit enter. Both SDK’s should appear in the console.
Render Extend offer buttons on your product display page
The Product Offer is used to display one or more protection plan offers directly on the product page and is the shopper’s first opportunity to add a warranty plan to the cart. Typically this will show 1-, 2-, and 3-year options for the same plan.
Adding the Extend offer element
Add an HTML element where you would like to place the Extend offer buttons. For highest conversion rates, we recommend placing it directly above the Add to Cart button. In most cases, the button is in the product-template.liquid, product.liquid, or product.form.liquid file, but if you have done previous work on your product page the it may be located somewhere else in your theme.
<div id="extend-offer">This is where the buttons will render</div>
Verify that the div has been added to the correct spot by temporarily adding some text, saving, and previewing your product page. Once you confirm that the text is showing in the correct spot, make sure to remove it!
Creating a custom product integration snippet
Inside your Shopify theme code editor create a new snippet called extend-product-integration. This is where you will leverage the Extend SDKs to display warranty offers on the product page that customers can add to their carts. As you will see, this file is effectively just a script that will be executed after your product display page is loaded.
Themes → Snippets → Add a new snippet
To ensure this snippet only runs when the Extend SDK is properly initialized, add the following code:
<script>
if (window.Extend && window.ExtendShopify) {
// Integration code will go here
}
</script>
Render the snippet on the same page you added the Extend product offer div by adding the following code to that page.
{% render 'extend-product-integration' %}
Rendering the Extend warranty offer buttons on the product page
Now that the snippet has been added, use the Extend.buttons.render()
function to render the offer buttons on the product page. This function takes two arguments:
- The ID of the element you added in the previous step (likely
#extend-offer
) - An object with a key of
referenceId
and a value of the selected productvariantId
// saves the selected variantId to a variable
var variantId = {{ product.selected_or_first_available_variant.id }}
// renders the Extend offer buttons for the product
Extend.buttons.render(
'#extend-offer',
{ referenceId: variantId }
)
Verify that the warranty buttons are rendering by previewing your theme and viewing a product that is active and enabled. If you don’t know a product that fits this criteria, you can find one in the merchant portal merchants.extend.com.
- Ensure are viewing a product that is matched and active
- Verify that your store is live (check the merchant portal, at the top of your screen)
- Verify that you are rendering the snippet on the page that contains offer div
Handle multiple product variants
In order to prevent a customer from accidentally purchasing the wrong warranty for a product, the warranty offers need to be updated every time a shopper selects a different variant for a product. This is done by passing the variantId
of the newly selected product to the Extend.setActiveProduct
function. Therefore, you will need to determine how your store updates the product page when a different product variant is selected. This is typically done by dispatching a JavaScript event or by manipulating the window location. Add an event listener to the page and invoke Extend.setActiveProduct()
with the newly selected variantId
.
Extend.setActiveProduct('#extend-offer', <VariantId>)
Verify that you are setting the correct variant by adding a console log right before the Extend.setActiveProduct()
function is called. This ensures you are passing the correct variantId
. You will also notice that if you change the variant on the page, the offer buttons will re-render.
Examples
Variant IDs are not accessible in the same way across all Shopify themes, but below are two of the most common scenarios we have seen:
Example 1: variantId
in the URL params
In the example below, the variantId
is available in the URL when the product variant changes. To set the active variant, you need to grab the variantId
from the URL and call Extend.setActiveProduct()
.
var productForm = document.querySelector('.product-form')
productForm.addEventListener('change', function() {
var urlParams = new URLSearchParams(window.location.search)
var variantId = urlParams.get('variant')
if (variantId) {
Extend.setActiveProduct('#extend-offer', variantId)
}
})
If you plan on enabling support for customers who use Internet Explorer 11, the above code would need to be rewritten in the following way, since URLSearchParams
would not be defined for them:
var productForm = document.querySelector('.product-form')
function getSearchQueryParam(searchString) {
// If user is on a modern browser, use its implementation of URLSearchParams
if (window.URLSearchParams) {
return new URLSearchParams(window.location.search).get(searchString)
}
var results = new RegExp('[?&]' + searchString + '=([^&#]*)').exec(window.location.search)
if (results == null) {
return null
} else {
return decodeURI(results[1]) || 0
}
}
productForm.addEventListener('change', function() {
var variantId = getSearchQueryParam('variant')
if (variantId) {
Extend.setActiveProduct('#extend-offer', variantId)
}
})
Example 2: variantId
in theme.js file
Add the Extend offer modal and add warranties to the cart
The Modal Offer is a modal rendered before navigating the customer to a new page and adding a product to cart, or as an opportunity on the cart page. In the example below, the offer modal appears after the customer added the product to the cart without selecting one of the offered protection plans.
Add an eventListener to the Add to Cart button
Select the Add to Cart button element on the product page using vanillaJS or jQuery and add an eventListener.
var addToCartButton = document.querySelector("button[name='add']")
addToCartButton.addEventListener('click', function(e) {})
In order to add the warranty to the cart or launch the offer modal, you need to prevent the default behavior of the Add to Cart button. You can do this by adding an event.preventDefault()
or event.stopImmediatePropagation()
inside the eventListener.
e.preventDefault()
or
e.stopImmediatePropagation()
Inside the Add to Cart eventListener, add ExtendShopify.handleAddToCart()
function. Make sure to select quantity
value from product form and add to ExtendShopify.handleAddToCart()
function.
addToCartButton.addEventListener('click', function(e) {
e.preventDefault()
var quantityEl = document.querySelector('[name="quantity"]')
var quantity = quantityEl && quantityEl.value
ExtendShopify.handleAddToCart('#extend-offer', {
quantity: quantity,
modal: true,
done: function() {
// call function to add your product here
},
})
})
To launch the offer modal if a warranty is not selected, set modal: true
. If you do not want to launch the offer modal, set modal: false
.
Resume Add to Cart function
Once the warranty has been added to the cart or the shopper has decided to not add a warranty (from the offer modal), you need to resume the Add to Cart function so that the product gets added to the cart. Inside the done
function, trigger your theme’s product form submit function. This can be done in a number of ways, so it is up to you to determine the best solution for your theme.
Standard Add to Cart flow (i.e form submission):
// select the form where the Add to Cart button is in.
var productForm = document.querySelector('.product-form')
// call the submit method on the form element to trigger the form submission
productForm.submit()
Add extended warranties to your cart
NOTE: If you’re integrating shipping insurance as well, proceed to run through Extend Shipping Insurance Integration guide from this point on.
The cart offer is the last chance your shoppers have to add an extended warranty before they checkout. Here you can display an offer button next to each eligible product in the cart that does not already have a protection plan associated with it.
Cart offer example:
Add the Extend cart offer element
Add an HTML element where you would like to place the Extend cart offer buttons. We recommend placing the element directly below each product in the cart. In most cases, that is in the cart.liquid or the cart-template.liquid file.
You need to add this button under each product in the cart that does not have a warranty. Find where the cart items are being iterated on in the template. Then set the quantity
and variantId
of the product to the cart offer div data attributes:
<div
id="extend-cart-offer"
data-extend-variant="{{ item.variant.id }}"
data-extend-quantity="{{ item.quantity }}"
></div>
Verify that the div has been added to the correct spot by temporarily adding some text, saving, and previewing your cart page. Once you confirm that the text is showing in the correct spot, make sure to remove it!
You also need to verify that the quantity
and variantId
are being passed into the cart offer div correctly. In your preview, navigate to your cart and inspect the page. You won’t be able to see the Extend cart offer buttons on the page, but you should see the HTML element.
Create custom cart integration snippet
Inside your Shopify theme code editor create a new snippet called extend-cart-integration. This is where you will call the Extend APIs to handle adding warranties to the cart.
Themes → Snippets → Add a new snippet
Render the snippet in the template where you added your Extend cart offer div (likely your cart page template file).
To ensure this snippet only runs when the Extend SDK is properly initialized, add the following code:
<script>
if (window.Extend && window.ExtendShopify) {
// cart integration code goes here
}
</script>
findAll
and hardRefresh
. findAll
will be used to select all of the cart offer divs on cart page. hardRefesh
ensures we do not run into any HTML caching issues when your cart page is refreshed after updates the cart have been detected.
var slice = Array.prototype.slice
function findAll(element) {
var items = document.querySelectorAll(element)
return items ? slice.call(items, 0) : []
}
function hardRefresh() {
location.href = location.hash
? location.href.substring(0, location.href.indexOf('#'))
: location.href
}
hardRefresh
we guarantee that all cart line item quantity updates are always reflected in the DOM across all browsers. As you will see below, this function will be invoked after adding a plan to the cart via a cart offer and after cart normalization updates have been made to the cart.
Gotcha: In order to hard refresh appropriately across all browsers, we need to use location.href = location.href, which does not work if there is a hash tag # in the URL. The hardRefresh function above safely strips hash tags from the URL. This means when the page reloads, the user will always land at the top of the page.
Render cart offer buttons
Call the findAll
helper method we added in the last step to find all the Extend cart offer divs. Here you need to pass in the ID of the Extend cart offer element (#extend-cart-offer
).
As you iterate through each item, pull out the variantId
and the quantity
from the #extend-cart-offer div data attributes.
var variantId = el.getAttribute('data-extend-variant')
var quantity = el.getAttribute('data-extend-quantity')
Use the warrantyAlreadyInCart()
function to determine if you should show the offer button.
You can access the Shopify cart object by declaring this variable at the top of your page:
var cart = {{ cart | json }}
if (ExtendShopify.warrantyAlreadyInCart(variantId, cart.items)) {
return
}
Then render the cart offer buttons using the Extend.buttons.renderSimpleOffer()
function.
Extend.buttons.renderSimpleOffer(el, {
referenceId: variantId,
onAddToCart: function(options) {
ExtendShopify.addPlanToCart(
{
plan: options.plan,
product: options.product,
quantity: quantity,
},
function(err) {
// an error occurred
if (err) {
return
} else {
// Effectively hard reloads the page; thus updating the cart
hardRefresh()
// For ajax carts invoke your cart refresh function
}
},
)
},
})
ExtendShopify.addPlanToCart
also takes a callback that can be used to refresh the cart to reflect the recently added warranty.
function (err) {
// an error occurred
if (err) {
return
} else {
// Effectively hard reloads the page; thus updating the cart
hardRefresh()
}
}
Verify the cart offer buttons are rendering correctly by previewing your theme and going to your cart page that has an active and enabled product in it. You should see the Extend cart offer button in the cart, and when you click it, it should launch the offer modal. When a shopper clicks this offer button, the modal described in the previous section will launch, and the shopper will be able to select which warranty plan he or she would like to purchase.
Cart normalization and quantity matching
As part of the checkout process, customers often update product quantities in their cart. The cart normalization feature will automatically adjust the quantity of Extend protection plans as the customer adjusts the quantity of the associated product. If a customer increases or decreases the quantity of products, the quantity for the related warranties in the cart should increase or decrease as well. In addition, if a customer has completely removed a product from the cart, any related warranties should be removed from the cart so the customer does not accidentally purchase a protection plan without a product.
To leverage cart normalization, you need to add the Extend normalize function to the cart integration script. First add the cart
variable:
var cart = {{ cart | json }}
Then add the ExtendShopify.normalizeCart
function and pass the Shopify Cart object to it:
ExtendShopify.normalizeCart({ cart: cart, balance: false }, function(err, data) {
if (data && data.updates) {
// Effectively hard reloads the page; thus updating the cart
hardRefresh()
}
})
ExtendShopify.normalizeCart
will return a promise that will give you the data
and err
object to check if the cart needs to be normalized. If the data
object exists and the data.updates
is set to true
, you will then call your function to refresh the cart page. Typically reloading the page will work for most Shopify cart pages.
Balanced vs unbalanced carts
Now that you have the normalize function in place, you need to decide if you want a balanced or unbalanced cart.
- Balanced cart: Whenever the quantity of a product with a warranty associated with it is increased, the quantity of the extended warranty sku associated with it will also increase to match.
- Unbalanced cart: Whenever the quantity of a product with a warranty associated with it is increased, the quantity of the extended warranty sku will remain the same, and it is up to the shopper to decide if he or she wants to add warranties to protect those new products.
Balanced and unbalanced carts can be toggled with the balance: true/false
property
Ajax cart normalization
Not using an AJAX cart? Feel free to skip ahead to the Styling section.
If you are using an Ajax cart, the page does not reload whenever an item’s quantity is updated. This means that in order to normalize an Ajax cart, you need to identify when the quantity of an item changes and then run the ExtendShopify.normalizeCart
function. Typically, changing the quantity of the items in the cart will trigger a function that will make an API call to Shopify to update the cart. You need to get this new updated Shopify cart object and pass it into the ExtendShopify.normalizeCart
function.
Define the cart integration code in a function, and then call that function at the bottom of your script. In this example, we defined the function to be called initializeCartOffer
:
Now dispatch an event whenever an item in the cart gets updated. To do this, first add an eventListener in your cart integration script.
Inside the eventListener do the following:
- Make an API call to Shopify to get the most updated cart object
- Reassign the cart variable to the
newCart
object you get from the callback of the API call - Call the function where you wrapped your Extend cart integration script in
window.addEventListener('normalizeCart', function() {
$.getJSON('/cart.js', function(newCart) {
cart = newCart
initializeCartOffer()
})
})
Now that you have the eventListener initialized, you need to find where in your code to dispatch a custom event. Find where in your Shopify theme the quantity of a cart item is updated and dispatch an event back to the cart integration script to pull in the new Shopify cart object.
Example:
In the example below, the quantity of a cart item is being updated from the updateCart()
function in the site.js file:
Ajax Side Cart Integration
Ajax side-carts are quite common, and the integration is similar to that of a regular Ajax cart.
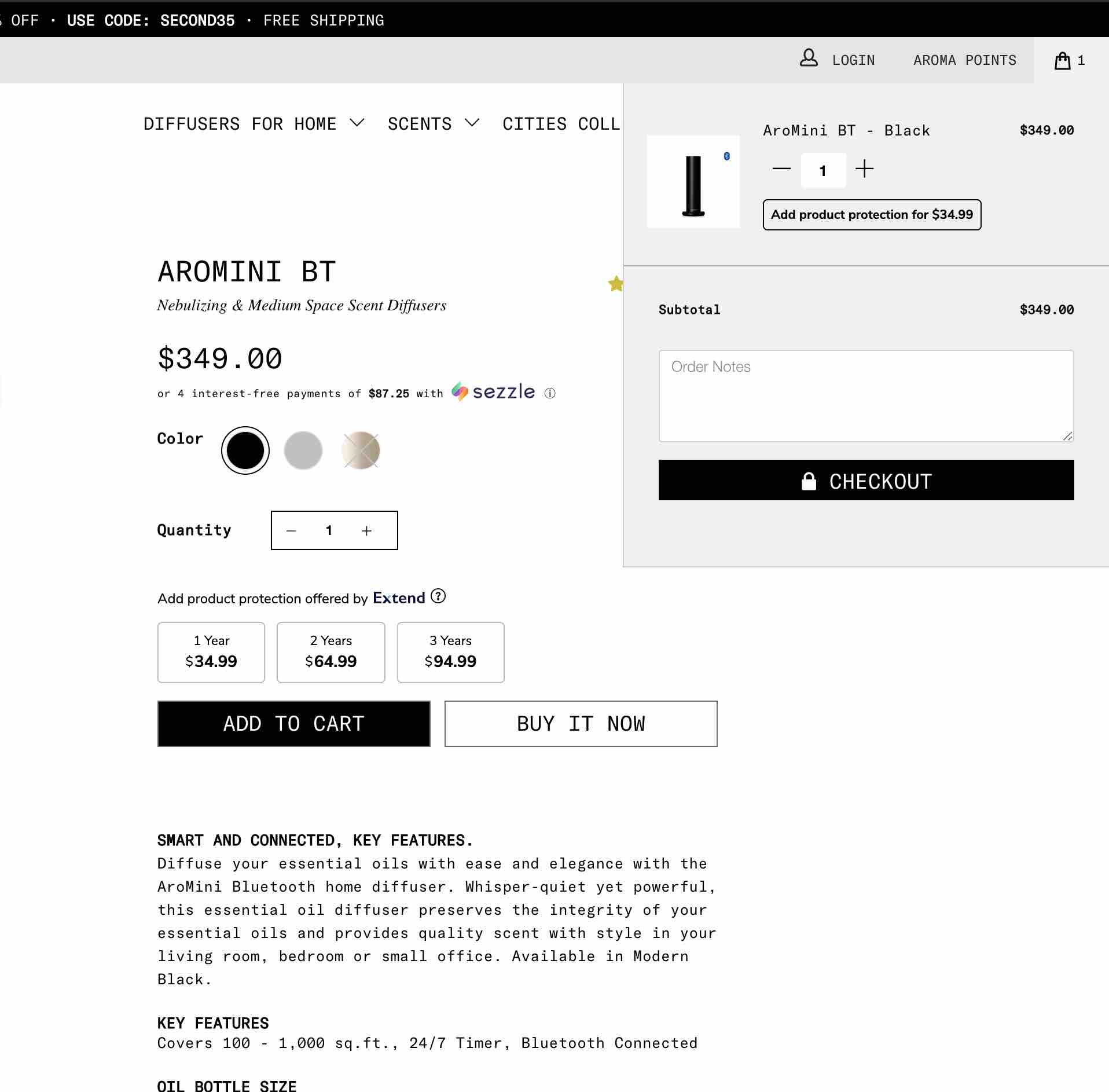
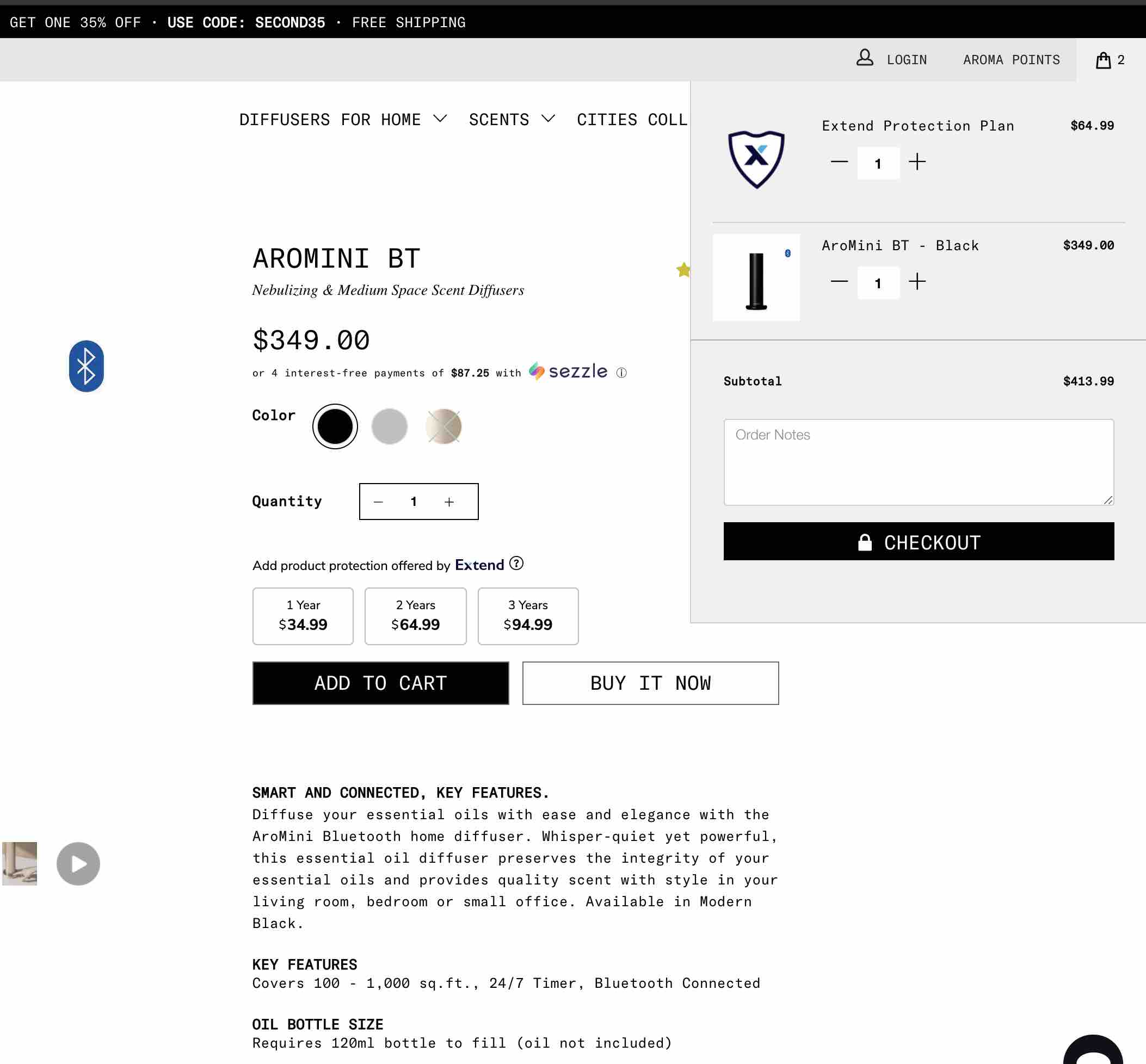
Add the Extend cart offer element
Add an HTML element where you would like to place the Extend cart offer buttons. We recommend placing it directly below each product in the cart. Typically this can be found in the ajax-cart-template.liquid file or in another similar template file.
You need to add this element under each product in the cart that does not have a warranty. Find where the cart items are being iterated on in the template. Then set the quantity
and variantId
of the product to the cart offer div data attributes:
<div
id="extend-cart-offer"
data-extend-variant="{{ variantId }}"
data-extend-quantity="{{ itemQty }}"
></div>
Create custom ajax side-cart integration snippet
Inside your Shopify theme code editor create a new snippet called extend-ajax-side-cart-integration. This is where you will call the Extend APIs to handle displaying offers on the product page and adding the warranties to the cart.
Themes → Snippets → Add a new snippet
Render ajax side-cart offer buttons
Copy the code from the extend-cart-integration snippet that you created and paste it into the extend-ajax-side-cart-integration snippet. This will render the cart offer buttons in your ajax-side cart.
Then add an eventListener to dispatch events from your themes.js file. This will help rerun the script whenever a product is added or the cart needs to be normalized.
window.addEventListener('refreshSideCart', function() {
$.getJSON('/cart.js', function(newCart) {
cart = newCart
initializeCartOffer()
})
})
Adding a warranty from an ajax side-cart
Whenever an extended warranty is added from the ajax side-cart, you need to rebuild your ajax side-cart with the new Shopify cart object as well as call the ajax-side-cart-integration script. This will add the warranty to the cart as well as remove the cart offer button from the product in your side-cart.
window.dispatchEvent(new CustomEvent('cartItemUpdated'))
If you plan on enabling support for customers who use Internet Explorer 11, the above code would need to be rewritten in the following way, since CustomEvent
would not be defined for them:
function createCustomEvent(eventName, params) {
// If user is on a modern browser, use its implementation of CustomEvent
if (window.CustomEvent) {
return new CustomEvent(eventName, params)
}
var options = params || { bubbles: false, cancelable: false, detail: null }
var evt = document.createEvent('CustomEvent')
evt.initCustomEvent(event, options.bubbles, options.cancelable, options.detail)
return evt
}
window.dispatchEvent(createCustomEvent('cartItemUpdated'))
In the example below we add our eventListener to allow us to run the function that builds the ajax side-cart. This eventListener will be ran from the custom dispatched event we sent in the previous example.
window.addEventListener('cartItemUpdated', function() {
Extend.buttons.instance('#extend-cart-offer').destroy()
$.getJSON('/cart.js', function(cart) {
cartUpdateCallback(cart)
})
})
Once the ajax side-cart is rebuilt, you may also need to dispatch an event back to the ajax-side-cart-integration snippet to allow for the script to be run again.
Ajax side-cart normalization
In order to normalize the ajax side‐cart, find where in your theme the ajax side-cart is rebuilt/updated when the quantity of a product is changed and dispatch a custom event to the same eventListener that was setup in your ajax-side‐cart-integration snippet.
Example:
Once our script is rerun and we determine we need to normalize the cart, we will dispatch an event to the extend-side-cart-integration file to allow for the ajax side-cart to be rebuilt/refreshed with the new Shopify cart object.
Styling the Warranty in the Cart
Most cart templates will iterate through a product’s details and display them on the cart page. They will also link to the product’s page from the thumbnail image and from the product title. In the case of the warranty, we recommend hiding the meta-data and disabling the links to the warranty product page so a customer cannot purchase a warranty without a matching product.
Disabling Links to Warranty Product Page
First, make sure you have an Extend warranty added to your cart. Next, navigate to the file where cart items are created. Typically, this will be the cart-template.liquid
file. Within the file, find the line of code that iterates through the items in the cart.
{% for item in cart.items %}
or
{% for line_item in cart.items %}
Within that for loop, find the elements where class=item-image
and class=item-title
. The class names might be slightly different, i.e. they may have cart-
prepended. Within the opening tags of both of those elements, add the following line of code:
{% if item.vendor == 'Extend' %} style="pointer-events: none;" {% endif %}
Or, if the element is referred to as a line_item
:
{% if line_item.vendor == 'Extend' %} style="pointer-events: none;" {% endif %}
This will conditionally disable the links on the warranty’s thumbnail image and its title. Reload the page and ensure the links are disabled!
Hiding Warranty Meta-Data
Within the same file, typically below the item-title
element, you’ll see a section of code that iterates through the product’s options
. That will look something like the following (depending on how the individual cart items are named):
{% for option in item.product.options %}
Above that line, there will be an conditional to check whether the product options should be displayed. Often it will look lik e the following:
{% unless line_item.variant.title contains 'Default'}
Add an or
statement to the conditional to check whether it’s an Extend warranty:
{% unless line_item.variant.title contains 'Default' or line_item.vendor == 'Extend' %}
Finally, you’ll see a section of code that lists the products properties. Look for an element with a class name that starts with product-details
. Within the opening tag of that element, you’ll see a conditional that looks something like the following:
{%if property_size == 0%} hide{% endif %}
Add or
statements to the conditional to check whether the property is of type Ref
, Extend.LeadToken
or Extend.LeadQuantity
:
{%if property_size == 0 or p.first == 'Ref' or p.first == 'Extend.LeadToken' or p.first == 'Extend.LeadQuantity' %} hide{% endif %}
The final product should look something like the following:
<tbody>
{% for line_item in cart.items %}
<tr class="cart-item" data-id="{{ line_item.id }}">
<td class="image">
<div
class="item-image"
{% if line_item.vendor == 'Extend' %}style="pointer-events: none;" {% endif %}
>
<a href="{{ line_item.url }}">
<img src="{{ line_item.image | img_url: 'small' }}" alt="{{ line_item.title }}" />
</a>
</div>
</td>
<td class="item-name">
<div
class="item-title"
{% if line_item.vendor == 'Extend' %} style="pointer-events: none;" {% endif %}
>
<a href="{{ line_item.url }}">
<span class="item-name">{{ line_item.product.title }}</span>
</a>
{% unless line_item.variant.title contains 'Default' or line_item.vendor == 'Extend' %}
<div class="wrap-item-variant">
{% for option in line_item.product.options %}
<span class="item-variant">{{ option }}: <span class="variant-title">{{ line_item.variant.options[forloop.index0] }}</span></span>
{% endfor %}
</div>
{% endunless %}
{% assign property_size = line_item.properties | size %}
{% if property_size > 0 %}
{%- for p in line_item.properties -%}
{%- unless p.last == blank -%}
<li class="product-details__item product-details__item--property {%if property_size == 0 or p.first == 'Ref' or p.first == 'Extend.LeadToken' or p.first == 'Extend.LeadQuantity' %} hide{% endif %}" data-cart-item-property>
<!-- NO FURTHER EDITS TO THIS SECTION OF CODE NEEDED -->
</div>
</td>
</tr>
</tbody>
That’s it! Give your page a refresh and ensure you can no longer see the warranty Reference Number and Title.
Support post-purchase warranty functionality
Extend provides a way for merchants to offer customers protection plans for products they have already purchased. In order to display post-purchase warranties to customers, they need to be added as leads. To learn more about creating leads, check out our documentation here. Once you have created leads, you can enable post-purchase offers in your store by following these two steps:
First, create a new snippet with the following title: extend-aftermarket-integration
.
Within that snippet, copy and paste the following code:
<script>
if (window.Extend && window.ExtendShopify) {
function getQueryParam(name) {
var results = new RegExp('[\?&]' + name + '=([^&#]*)').exec(window.location.href)
if (results == null) {
return null
}
return decodeURI(results[1]) || 0
}
var leadToken = getQueryParam('leadtoken')
if (leadToken) {
Extend.aftermarketModal.open({
leadToken: leadToken,
onClose: function(plan, product, quantity) {
if (plan && product) {
ExtendShopify.addPlanToCart(
{
plan: plan,
product: product,
leadToken: leadToken,
quantity: quantity,
},
function() {
// You can insert a custom callback if you don't want to redirect to the cart page
window.location = '/cart'
},
)
}
},
})
}
}
</script>
Next, in your theme.liquid
file, add the following line of code before the closing <head>
tag:
{% render 'extend-aftermarket-integration' %}
That’s it! You have enabled post-purchase offers for customers added as leads.
Final review
Congratulations, you have finished integrating the Extend Client SDK into your store! Before you publish your theme and start selling extended warranties, please make sure to go through the integration checklist, which you can find here.
ExtendShopify API reference
Introduction
Welcome to the ExtendShopify
API reference! We’re happy that you’ve decided to partner with us and leverage our Shopify SDK. This reference details the functions available to you via the ExtendShopify
SDK interface and should be used in conjunction with our Extend Shopify Integration Guide
. If you haven’t already done so, be sure to create your store and add the Extend base SDK and Extend Shopify SDK scripts to your store’s theme.liquid
file.
Table of Contents
- Add plan to cart (
#addPlanToCart
) - Normalize cart(
#normalizeCart
) - Handle Add to Cart(
#handleAddToCart
) - Warranty already in cart(
#warrantyAlreadyInCart
)
ExtendShopify.addPlanToCart(options: AddToCartOptions, callback: function)
This function adds an Extend warranty plan to the cart. However, it should only be used within a callback that is provided to an Extend base SDK function that returns an Extend Plan and Product. Important Note: If you are looking to add a product and its associated warranty to the cart, please see #handleAddToCart
instead.
ExtendShopify.addPlanToCart({ plan, product, quantity }, callback)
Extend.buttons.renderSimpleOffer
and
Extend.aftermarketModal.open
Attributes
Attribute | Data type | Description |
---|---|---|
addToCartOptions required |
object | AddToCartOptions |
callback optional |
function | Callback function that will be executed after the item is added to the cart |
AddToCartOptions Object
Attribute | Data type | Description |
---|---|---|
plan required |
object | Extend plan object to be added to the cart |
product required |
object | Product associated with the warranty plan |
quantity optional |
number | Number of plans to be added to the cart (defaults to one) |
leadToken optional |
string | Token used for post purchase offers |
Interfaces
interface addPlanToCartProps {
opts: AddToCartOpts
callback?: Callback
}
interface AddToCartOpts {
plan: PlanSelection
product: Product
quantity: number
leadToken?: string
}
ExtendShopify.normalizeCart(options: NormalizeCartOptions, callback: function)
This function accepts and updates the Shopify cart object to ensure that the line item quantity of a warranty is not greater than the line item quantity of its associated product and returns an object containing the updated cart and cart updates. Therefore, this function should be executed every time the cart is updated in order to ensure a user cannot buy a warranty for a product not in the cart. While optional, a callback should almost always be passed as a second argument. This callback will be executed after the cart normalizes and should therefore be used to update the quantity input selectorson the page with their updated values, typically via a hard refresh.
ExtendShopify.normalizeCart({ cart: cart, balance: false }, function(err, data) {
if (data && data.updates) {
hardRefresh()
}
})
Attributes
Attribute | Data type | Description |
---|---|---|
normalizeCartOptions required |
object | NormalizeCartOptions |
callback optional |
function | Callback function that will be executed after the normalizeCart function is invoked (Typically refreshes the cart) |
NormalizeCartOptions Object
Attribute | Data type | Description |
---|---|---|
cart optional |
object | Shopify cart object to be normalized |
balance optional |
boolean | When set to true warranty quantity will equal the associated product quantity |
Interfaces
interface NormalizeCartProps {
normalizeCartOptions: NormalizeCartOptions
doneCallback: Callback
}
interface NormalizeCartOptions {
balance?: boolean
cart?: Cart
update?: boolean | UpdateFunction
}
Normalize cart response object
Attributes
Attribute | Data type | Description |
---|---|---|
cart | object | Normalized Cart Object |
updates | object or null | Object containing the updated variantId ’s and their updated quantities |
Interfaces
interface NormalizeCartResult {
cart: Cart
updates: CartUpdates | null
}
interface CartUpdates {
[variantId: string]: number
}
interface Cart {
token: string
note: string
attributes: { [key: string]: string }
total_price: number
total_weight: number
item_count: number
requires_shipping: boolean
currency: string
items: CartLineItem[]
}
ExtendShopify.handleAddToCart(element: string, options: HandleAddToCartOpts)
This function will check the Extend offer buttons for a selected plan. If a plan is selected, the function will add the plan to the cart and then execute a callback provided in the HandleAddToCartOpts
object. If a plan is NOT selected, the function will check the HandleAddToCartOpts
to determine whether or not to render the Extend offer modal. The done
callback provided will be executed after the user adds a warranty, clicks ‘No, thanks’ or closes the modal. Use this callback to call your stores add to Cart function or your product submit form.
ExtendShopify.handleAddToCart('#extend-offer', {
quantity: quantity,
modal: true,
done: function() {
// callback logic to add the product to the cart
},
})
Attributes
Attribute | Data type | Description |
---|---|---|
element required |
string | The ID of the container element used to render the Extend offer buttons |
HandleAddToCartOpts required |
object | HandleAddToCartOpts |
HandleAddToCartOpts Object
Attribute | Data type | Description |
---|---|---|
modal optional |
boolean | Determines whether to render the modal if no plan is selected (defaults to true) |
quantity optional |
integer | Quantity of warranties to add to the cart (defaults to one) |
done optional |
function | Callback executed after the warranty business logic |
Interfaces
interface HandleAddToCart {
element: string | Element
opts: HandleAddToCartOpts
}
interface HandleAddToCartOpts {
modal?: boolean
quantity?: number
done?: Callback
}
ExtendShopify.warrantyAlreadyInCart(variantId: string, cartItems: CartItem[])
This function accepts a Shopify product variantId
and the Shopify cart items
array. The function iterates through the Shopify cart items and returns a boolean indicating if there is already a warranty in the cart for that product variantId
. This function is almost always used on the cart page to determine whether or not to render a cart offer button for a line item in the cart.
var cart = {{ cart | json }}
if (ExtendShopify.warrantyAlreadyInCart(variantId, cart.items)) {
return;
} else {
// render a cart offer button
}
Attributes
Attribute | Data type | Description |
---|---|---|
variantId | string | This Shopify variantId of the product to be checked for a warranty |
cartItems | array | The array of cart items stored on the Shopify cart items property |